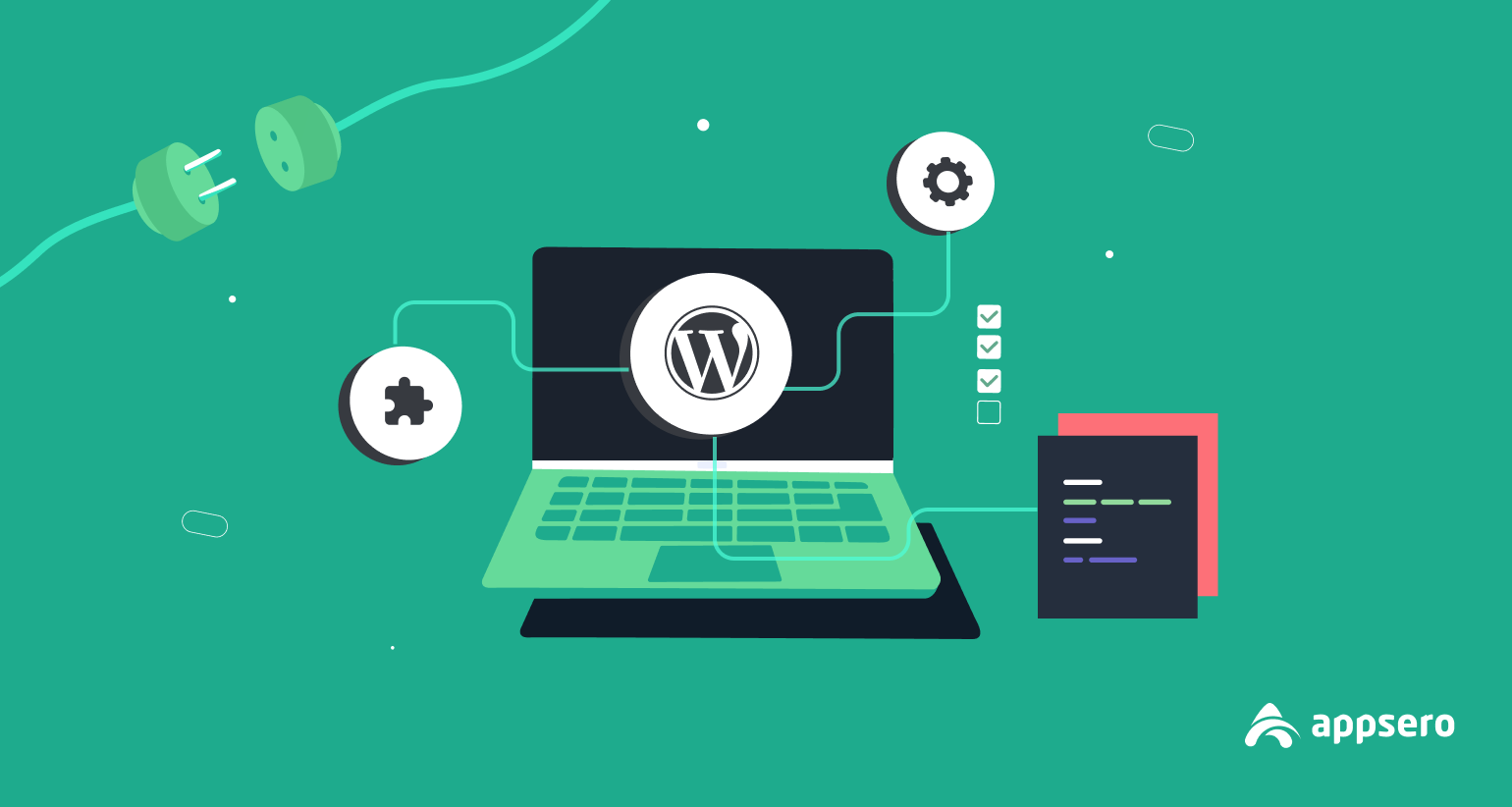
WordPress Plugin Development Best Practices You Should Follow
The WordPress ecosystem is growing bigger each day with numerous plugins and themes. Currently, it has 60K+ plugins on the official WordPress.org repository.
If you’re planning to add your plugin to that number, make sure to follow the best coding practices to get approval.
WordPress is an open-source platform that emphasizes the contribution and benefits of the WordPress community across the globe. That’s why you need to follow a set of rules to match the global standard.
In this article, we are going to show you how to develop a plugin effectively by following WordPress plugin development best practices.
Before that, let’s have a quick look at the key stages of WordPress plugin development.
What are the Key Stages of WordPress Plugin Development
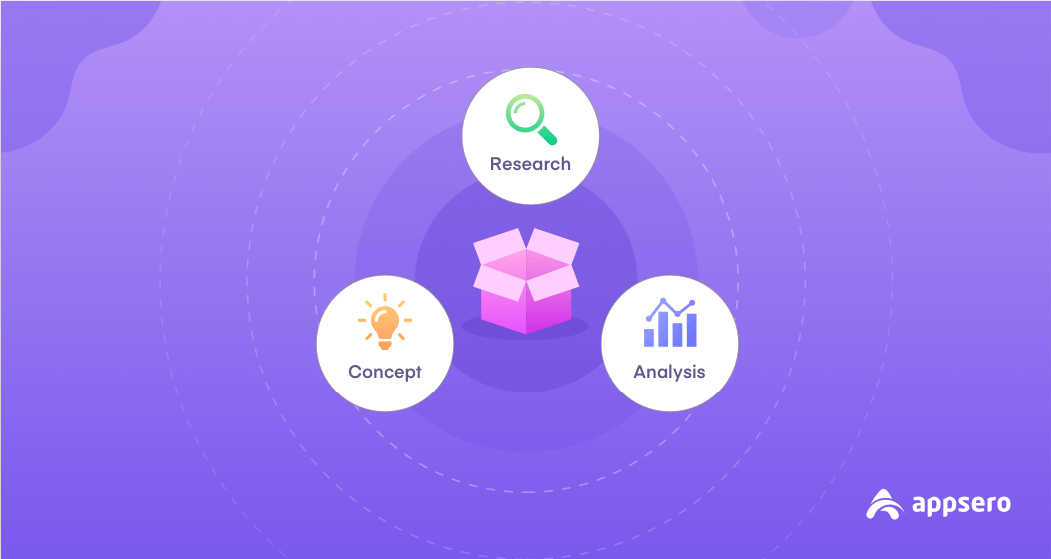
Before you plan to create a plugin, make sure it has utility in the WordPress eco-system. Prepare your basics first, then start coding accordingly. You need the answers to the following questions during the R&D process –
- Do WordPress users really need this plugin?
- What will be the unique features of your plugin?
- How these features can help the users?
- What will be the design for the front end?
- How will it integrate with necessary plugins and services?
Once you are sure you know what you’re doing, go on to make a WordPress plugin. The key stages of WordPress plugin development are as follows –
- Fix a name and consider trademark issues
- Create a folder and structure for the plugin
- Add the file header to your plugin
- Write the code to make your plugin functional
- Deploy your plugin to WordPress.
Also, you can read this blog to learn How to Create WordPress Plugins within 5 Steps. You need to submit your plugin to the WordPress review team before it finally becomes visible to everyone.
Know WordPress.org Review Team Before Deploying Your Plugin
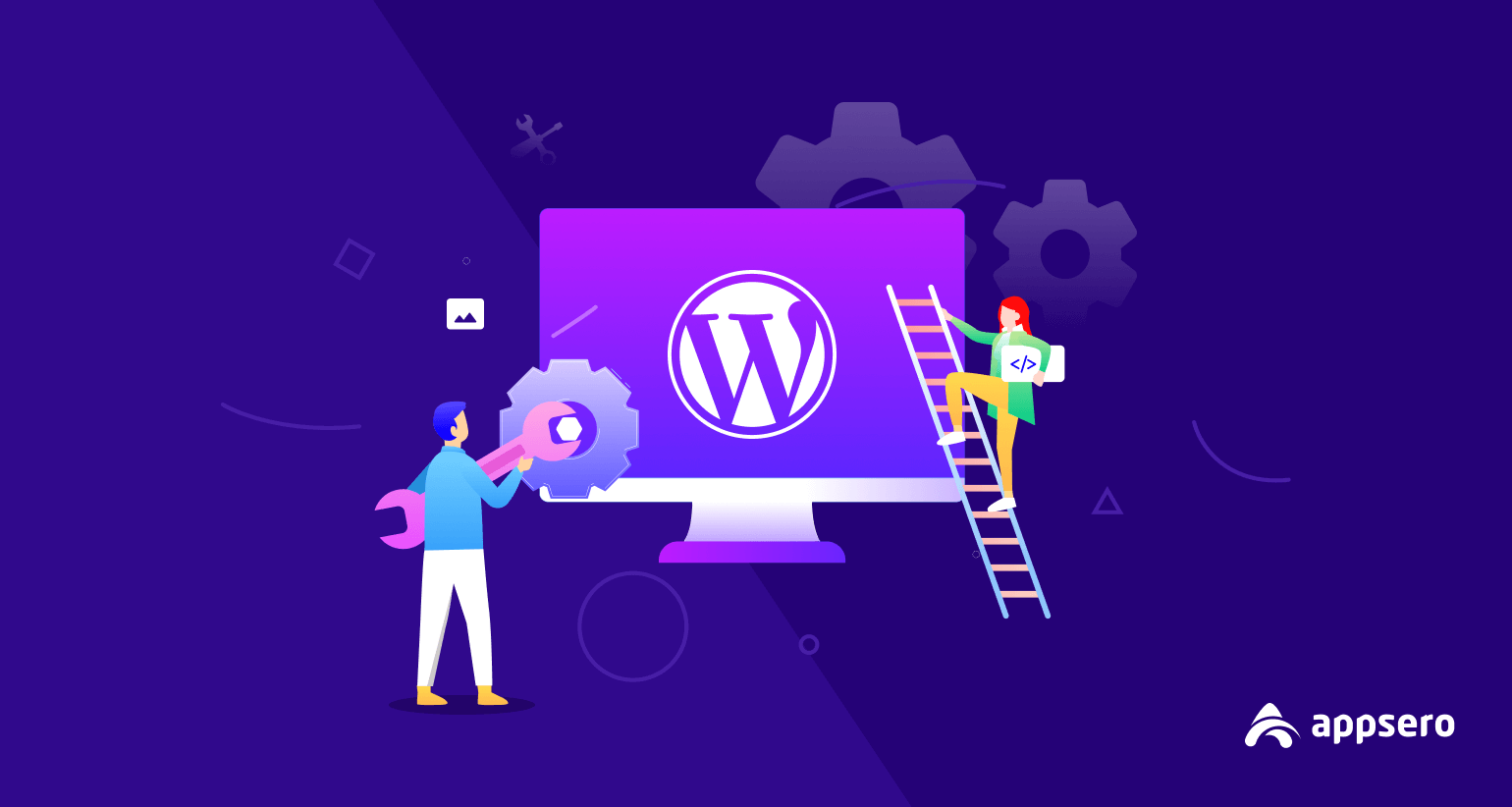
Each WordPress plugin undergoes a review process before it appears publicly on the WordPress.org official plugin directory. The review team comprises a group of experts who have vast experience in WordPress development. Currently, the review team has four valuable members. They voluntarily review the plugins using official tools according to the Plugin Reviewer Handbook.
They also investigate guideline infringements, security issues, and developers’ behavior across the WordPress community, including support forums, WordCamps, and reviews. You can pass through these gatekeepers only if you maintain the Coding Standards. That’s why you need to follow the best practices when developing a WordPress plugin.
12+ WordPress Plugin Development Best Practices
All your time and efforts will go in vain if your plugin doesn’t get approval from the review team. That’s why you need to follow WordPress development best practices when creating a plugin. You’re most likely to pass through the filtering process by following these steps minutely.
1. Create a Well-defined Strategy
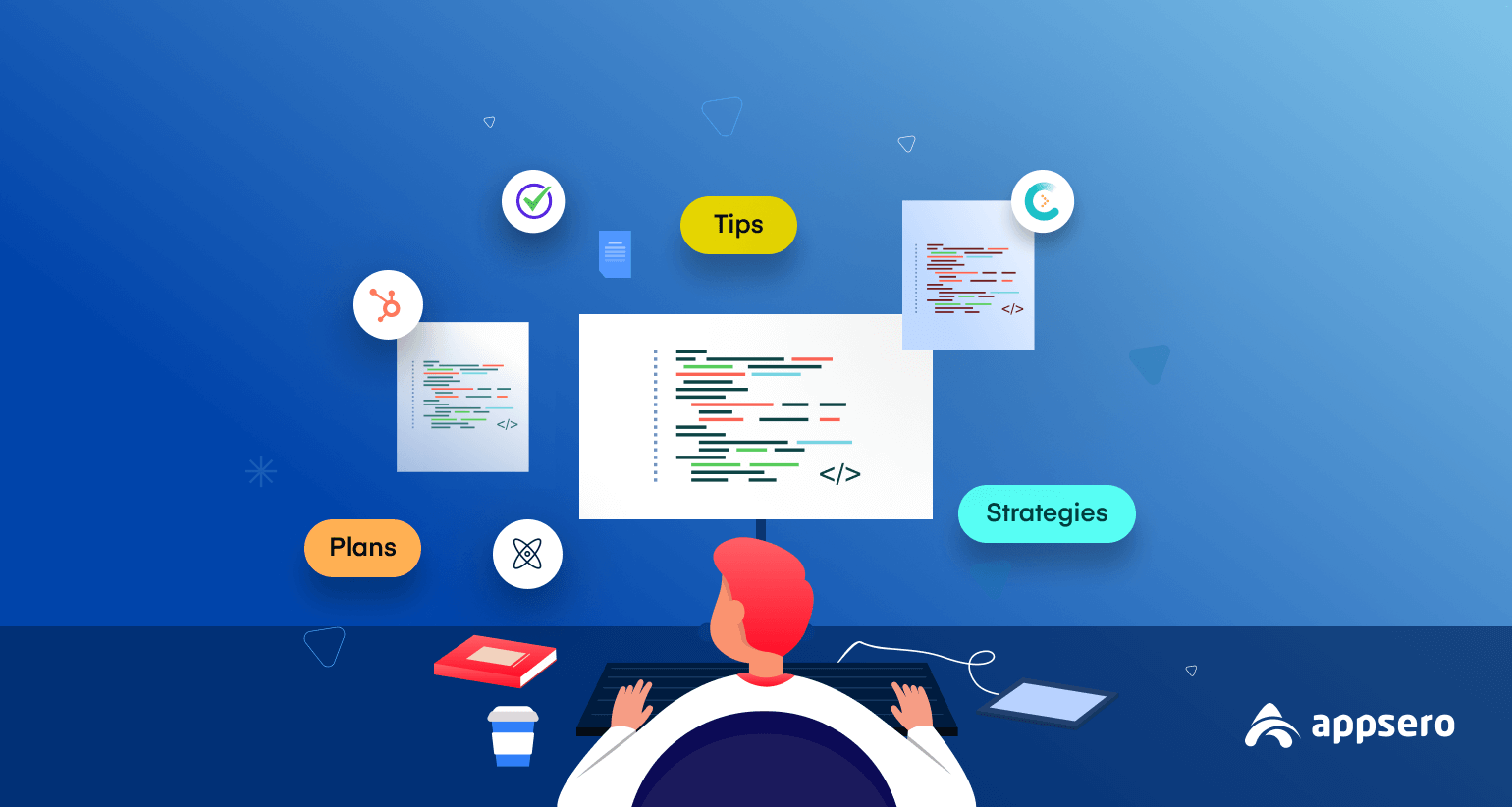
Without a proper plan and vision, the whole development process will result in a big zero. Find the pain points of your target audience and offer effective solutions for them.
You can only bring a new plugin if the already existing plugins aren’t enough to solve the problems you’re addressing. Nobody is going to clutter his website with your plugin If you fail to add value to their product.
So, it’s important to introduce a plugin that has unique selling points and is easily marketable. It should enhance a website’s accessibility and do everything in simpler ways. Only a well-defined strategy can help you create and market a plugin successfully.
2. Follow WordPress Coding Standards
There are thousands of WordPress developers worldwide. They need to follow a set of rules to avoid common errors, enhance code readability, and maintain consistency. Anyone from any corner of the globe should understand what’s written in a code snippet. It’s possible only if everyone follows the same WordPress Coding Standards.
WordPress has a set of language-specific and accessibility standards. They define what to do and what not to do when you’re working with CSS, HTML, JavaScript, and PHP codes. You have to abide by these standards to create a plugin that caters to the needs of the WordPress community.
3. Use Namespacing Carefully
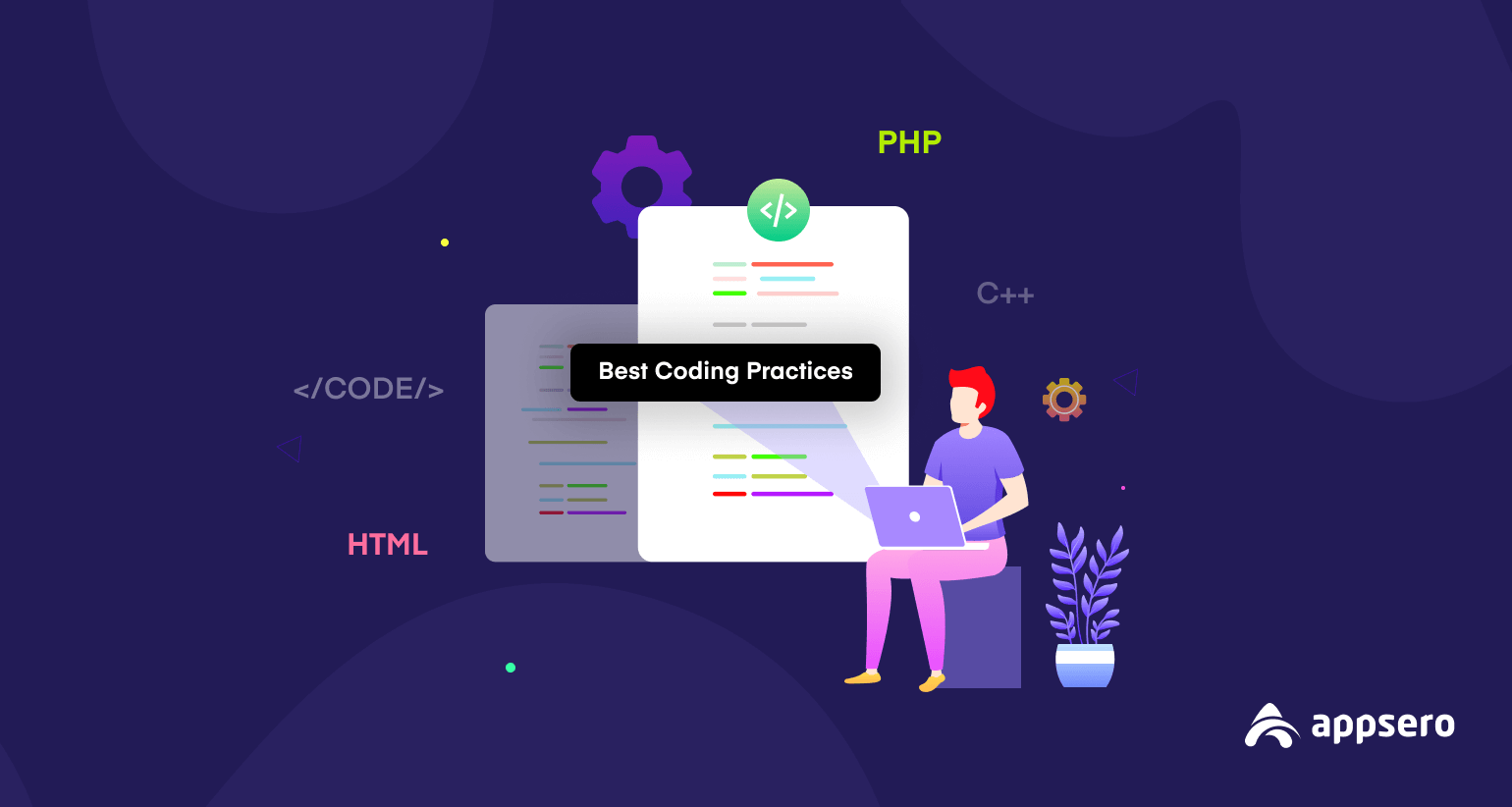
Whenever you add functions, variables, and classes to your plugin it gets thrown in the worldwide namespace. If the global namespace already has the same function written by someone else, it will conflict and show an error.
That’s why you need to add a unique prefix to your functions, variables, and classes. Prefixes help your plugin avoid overwriting issues.
For example, if your plugin’s name is WP Test Plugin, all your functions and variables should look like the following –
wp_test_plugin_some_function()
or $wp_test_plugin_my_variable
.
You can also shorten the plugin’s name by keeping only the first letters. WP Test Plugin would be ‘WTP’ in that case.
4. Make Security a Top Priority
Website security is one of the most important things for every website owner. Your plugin is less likely to get any user if it fails to earn their trust. So, invest more time in ensuring the security standard of your plugin. Give importance to the following sanitized inputs and escape outputs –
- esc_url
- esc_url_raw
- esc_html
- esc_textarea
- esc_attr
- wp_filter_kses
- wp_insert_post
- esc_textarea
- $wpdb->update
- $wpdb->insert
Also Read: WordPress Security Best Practices: 8 Focusing Strategies to Look out
5. Use Nonces for Verification
WordPress nonces refer to one-time tokens that secure the URLs and forms from unwanted actions. It works as a unique identifier for WordPress users when they take actions like deleting or previewing a post. So, use nonce within the WordPress environment to validate a specific action.
For example, when you want to delete a post, the URL generates a nonce token to verify whether the request is genuine or not. See the image below for reference. Hover your mouse on the Trash option it will show you the nonce token.
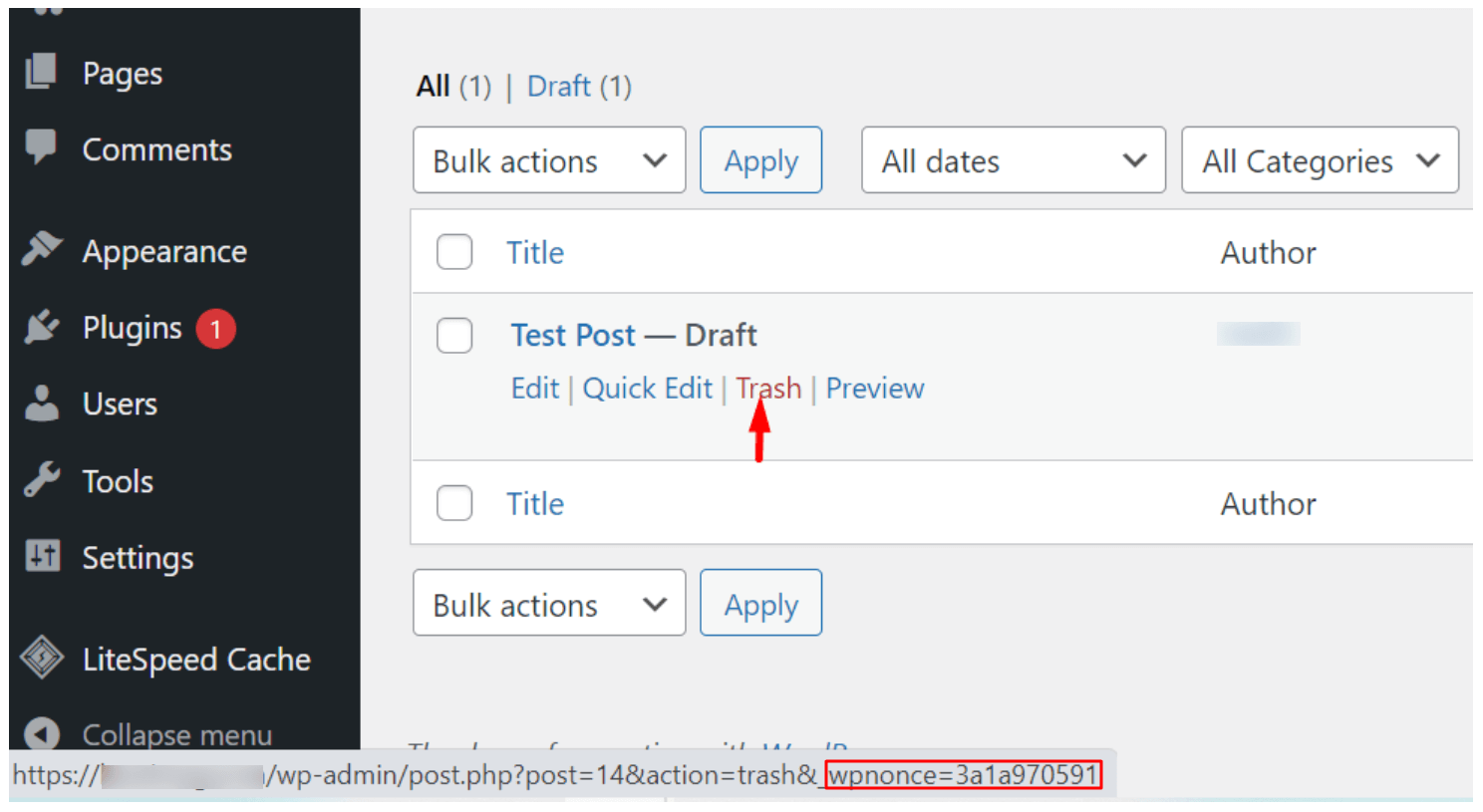
If this request comes from a fake source, it won’t have the wpnonce part at the end. That keeps you safe from unauthorized actions.
6. Start with Boilerplate Code
Creating a new plugin from scratch takes a lot of time and effort. You can start working with a boilerplate. Boilerplate is a foundation for plugin development. It provides precise and consistent guidelines for WordPress developers. An advantage of using a familiar boilerplate is it allows other people to contribute to your plugin easily.
7. Use WP_DEBUG Mode
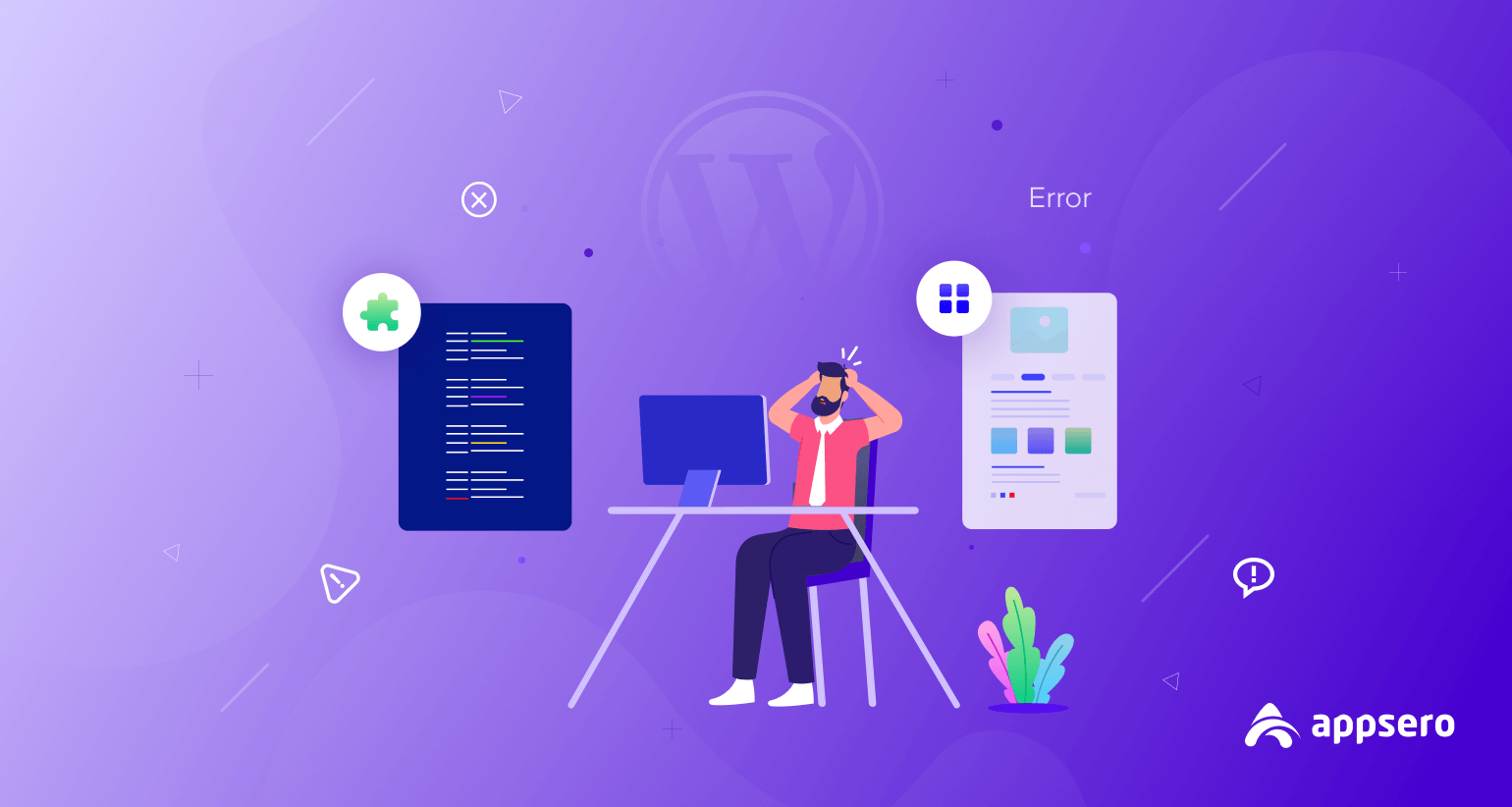
Turn the WP_DEBUG mode on when working with WordPress. It will find out the errors you make throughout the development process. You can enable the debugging mode by placing the following code snippet in your wp-config.php file.
define( 'WP_DEBUG', true );
define( 'WP_DEBUG_LOG', true )
Turn the WP_DEBUG off once the development process is off. Otherwise, it may show you PHP notices or other minor error notifications that don’t affect a plugin seriously. We suggest disabling the debugging mode in live environments.
8. Maintain a Clear Folder Structure
Maintaining a clear and well-organized folder structure is a must for WordPress plugin development. It is also helpful for the developer as it shows the entire workflow.
Don’t forget to place different types of files in the designated folders. For example, keep your images inside the /images folder, not anywhere else.
Here’s a sample folder structure for your plugin –
/plugin-name
plugin-name.php
uninstall.php
/languages
/includes
/admin
/js
/css
/images
/public
/js
/css
/images
Make sure your root directory has the plugin-name.php file and the uninstall.php file. Maintain proper hierarchy between all subfolders.
9. Add the Readme.Txt File
Your plugin can’t pass through the review team without a complete readme.txt file. It’s essential to facilitate the plugin’s distribution process. A readme file contains the following pieces of information:
- Name of the plugin
- Name of the contributors
- Required PHP version
- Required WordPress version
- Tags
- License information, and more.
Let’s have a look at the following readme.txt file as a sample.
=== Plugin Name ===
Contributors: (this should be a list of wordpress.org userid's)
Donate link: https://example.com/
Tags: tag1, tag2
Requires at least: 4.7
Tested up to: 5.4
Stable tag: 4.3
Requires PHP: 7.0
License: GPLv2 or later
License URI: https://www.gnu.org/licenses/gpl-2.0.html
Here is a short description of the plugin. No markup here.
You can add a short description (less than 150 characters) of the plugin at the end of the text. Anything longer than this limit will get cut off. But, you can add more information about various aspects of the plugin in the next Description section of the text file.
10. Focus on Internationalization
WordPress is available in each part of the world where the internet is available. Why will you miss out on this global audience?
So, emphasize the importance of internationalizing your plugin for a wider audience. Make your strings translatable using the gettext libraries. You can learn more from this WordPress guide on internationalization.
11. Include Only What’s Necessary
Don’t go overboard with your codes. Load only what you require to make the plugin functional and useful for your users. Only use stylesheets, JavaScripts, and other code snippets that serve a specific purpose.
12. Use Tools & Services When Needed
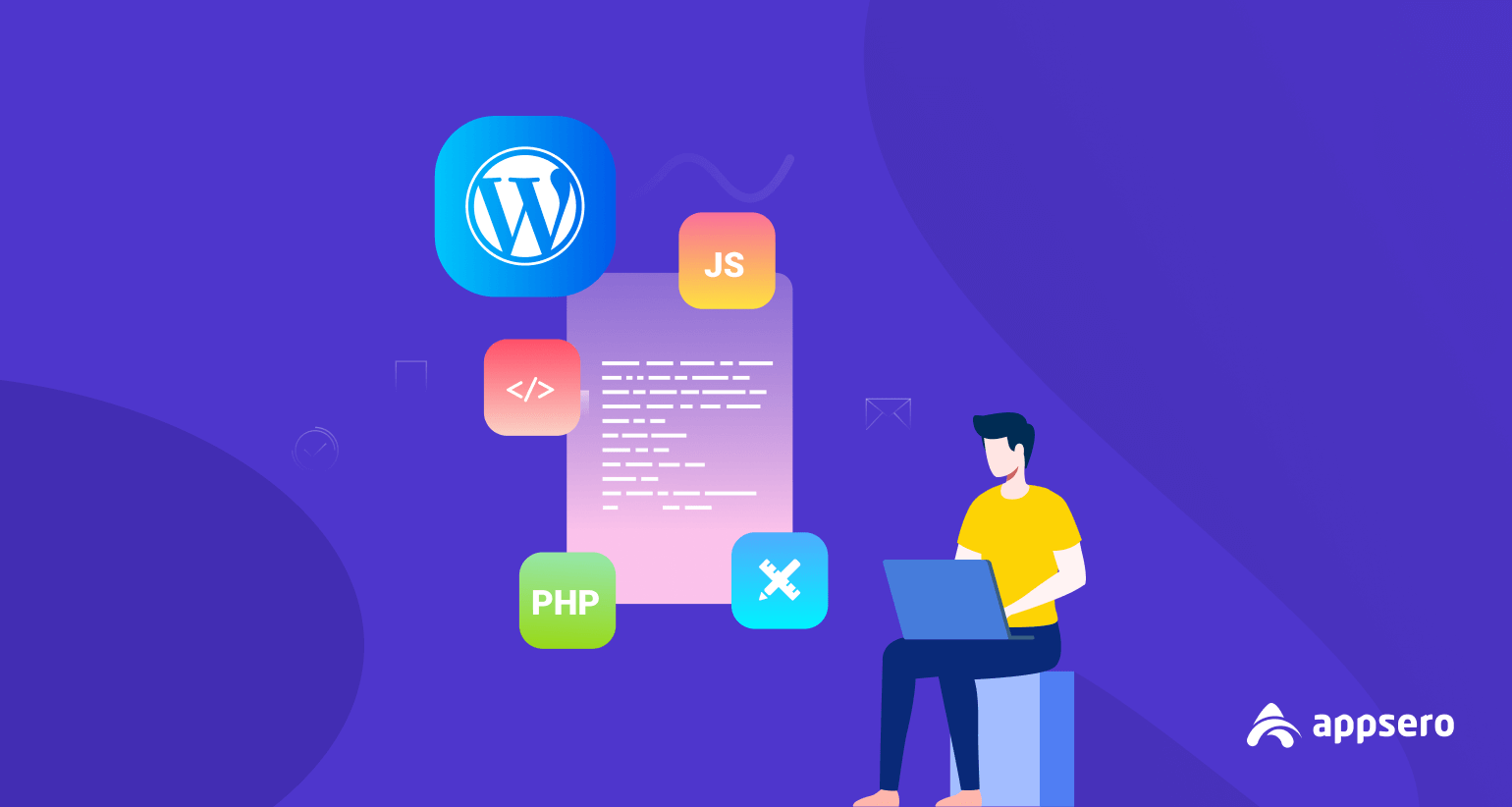
You can use WordPress plugin development tools to develop your plugin in easier ways.
A text editor to apply the codes, an FTP client to transfer and share files, and a staging environment. These three tools and services you need to use when developing WordPress plugins.
You can adopt agile development methods throughout the development stages to speed up the deployment process.
We suggest measuring the condition of your plugin with software testing tools like Codeception. You can test your product against the standard coding standards and determine the nature of the user experience with this tool.
Bonus Tips – Common WordPress Development Mistakes to Avoid
As a beginner-level developer, you may make hundreds of mistakes. Some mistakes are critical whereas some others happen for trivial reasons. WordPress developers usually make the following common mistakes.
- Turning the debugging mode off
- Paying less attention to SQL injection risks
- Not complying with rules set by WordPress
- Finding quick solutions from the web
- Using unnecessary CSS and JavaScript files
- Failing to reap the benefits of WordPress Core Functionality
- Not staying updated on the latest technologies
- Failing to manage licenses properly
You can also read the details from this blog ➡ 10 Common Mistakes to Avoid While Developing WordPress Plugins and Themes.
FAQs About WordPress Plugin Development Best Practices
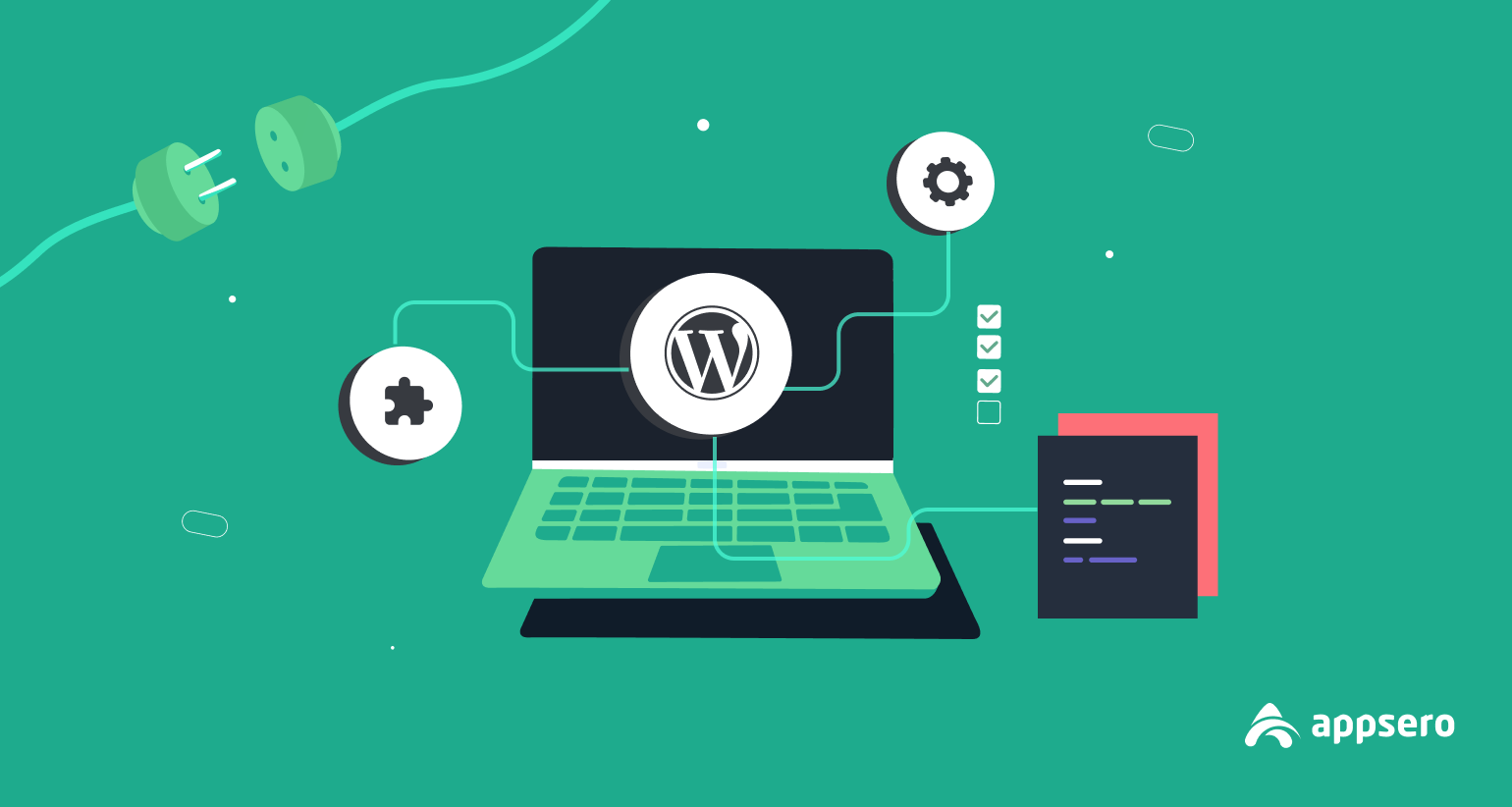
Is WordPress plugin development easy?
A WordPress plugin is both easy and difficult to make based on the functionality and features you want to add. You can create a plugin with a single PHP line, but that can do nothing significant. But, it’s not easy to build a feature-rich WordPress plugin.
How long it takes to make a WordPress plugin?
There’s no fixed period of time for developing a WordPress plugin. It can take from one day to one month or more based on the complicacy. But, it’s a continuous process. You need to add new features and bug fixes to keep the plugin relevant and functional.
How much I can earn by selling a WordPress plugin?
That depends on a lot of factors. If you have a large customer base who uses your plugin regularly you can earn even 100K USD per month. And, if your plugin has zero pro users, your earnings will be 0 USD. So, there’s no clear answer to this question.
Can I copy and sell plugins made by others?
Yes, legally there are no restrictions. WordPress products are licensed under GNU General Public License. It allows anyone to use and distribute WordPress plugins without the owner’s consent. These types of unauthorized plugins are called Nulled WordPress Plugins.
How to upload a Plugin to the WordPress Repository?
Adding plugins to the WordPress repository is a 5 steps process. Take a quick look at the list first.
Step 0: Get a Clear Idea of Plugin Structure
Step 1: Follow the Community Guidelines
Step 2: Set a Unique Plugin Name
Step 3: Validate the Readme File
Step 4: Submit Your Plugin for Approval
Step 5: Store Your Plugin in WordPress Subversion (SVN) Repository
Learn the details from this blog: How to Upload Plugin to WordPress Repository: Step by Step Guide for First-Timers.
Sell Your Plugin & Manage Licenses with Appsero
Now you know how to develop a WordPress plugin in the best ways. What’s next? If you plan to sell your plugin and manage licensing and deployment from a central hub, Appsero is here for you. You can sell your plugin, generate license keys, and gather detailed reports from a dedicated dashboard.
You can even sell a bundle of plugins and themes using Appsero. It is compatible with WooCommerce, Easy Digital Downloads, FastSpring, Paddle, and more. So, develop an error-free plugin today and start selling it with Appsero.
Subscribe To Our Newsletter
Don’t miss any updates of our new templates and extensions
and all the astonishing offers we bring for you.